Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- Hibernate
- 자바서블릿
- 대량쿼리
- 프로젝트생성
- jQueryUI
- JQuery
- JPQL
- javaservlet
- 페이징
- calendar
- fetchjoin
- 제네릭
- jQuery값전달
- joinfetch
- jscalendar
- values()
- namedQuery
- 스프링데이터흐름
- 벌크연산
- javascriptcalendar
- 제너릭
- LIST
- jQuery값전송
- paging
- Generic
- fullcalendar
- JPA
- 페치조인
- springflow
- 엔티티직접사용
Archives
- Today
- Total
가자공부하러!
네이버 파이낸셜 코테(2021-08-28) 본문
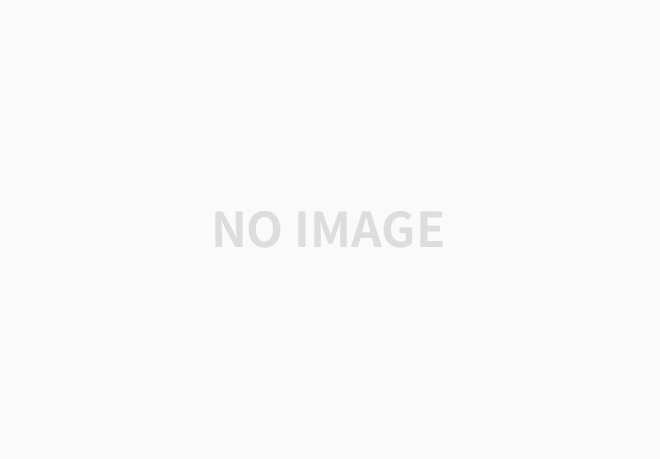
// 워드 머신은 정수 스택에 대해 일련의 간단한 작업을 수행하는 시스템입니다.
// 처음에는 스택이 비어 있습니다.
// 작업 순서는 문자열로 제공됩니다. 작업은 단일 공백으로 구분됩니다.
// 아래는 예시입니다. 다음 작업을 지정할 수 있습니다.
// - 정수 X(0~ 2^20-1) : 머신은 X를 스택에 저장합니다.
// - POP : 머신은 스택의 최신값을 제거합니다.
// - DUP : 머신은 스택의 최신값과 같은 값을 스택에 추가합니다.
// - + : 머신은 스택의 최근 두 개의 값을 꺼내서 더한 결과값을 스택에 추가합니다.
// - - : 머신은 스택의 맨 위 두 개의 값을 꺼내서 첫 번째 뺀 값에서 두 번째 것을 뺀 결과값을 스택에 추가합니다.
// 모든 작업이 수행된 후에 머신은 스택의 맨 위 값을 리턴합니다.
// 머신은 20-bit 부호없는 정수를 처리합니다.
// 덧셈의 오버플로나 뺄셈의 언더플로는 오류를 일으킵니다.
// 또한 머신은 스택에 실제로 포함된 것보다 더 많은 숫자가 스택에 있을 것으로 예상되는 작업을 수행하려고 할 때 오류를 보고합니다.
// 또한 모든 작업을 수행한 후 스택이 비어 있으면 시스템이 오류를 보고합니다.
if (S.contains("aaa")) {
return -1;
}
char[] chars = S.toCharArray();
int notACount = 0;
int currentACount = 0;
for (char aChar : chars) {
if ('a' != aChar) {
notACount++;
} else {
currentACount ++;
}
}
int totalACount = (notACount + 1) * 2;
return totalACount - currentACount;
2번
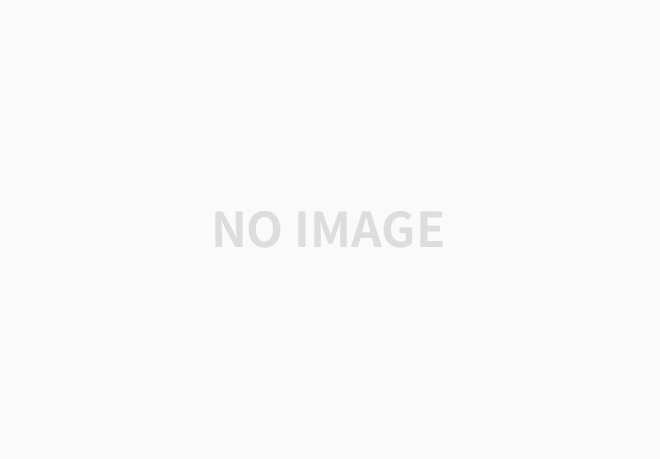
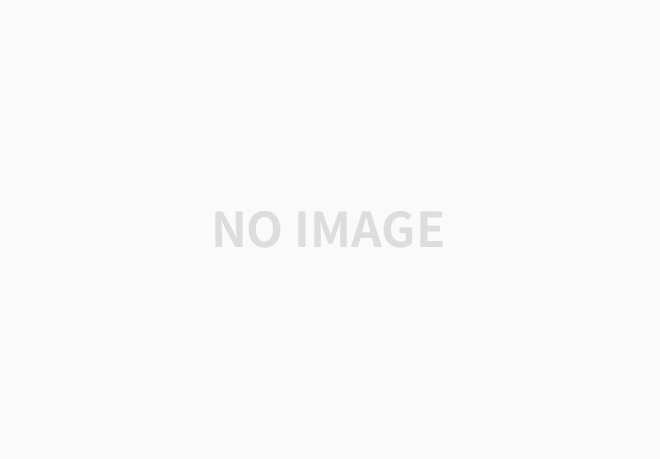
// you can also use imports, for example:
// import java.util.*;
import java.util.*;
// you can write to stdout for debugging purposes, e.g.
// System.out.println("this is a debug message");
class Solution {
public int solution(String S) {
Stack<Integer> stack;
try {
stack = operateWordMachine(S);
} catch (EmptyStackException | StackOverflowError | NumberFormatException e) {
return -1;
}
return stack.pop();
}
private Stack operateWordMachine(String S) {
Stack<Integer> stack = new Stack();
String[] sequence = S.split(" ");
for (String command : sequence) {
switch (command) {
case "POP" : stack.pop(); break;
case "DUP" : stack.push(stack.peek()); break;
case "+" : stack.push(stack.pop() + stack.pop()); break;
case "-" : stack.push(stack.pop() - stack.pop()); break;
default :
int number = Integer.parseInt(command);
if (number > 1_048_575) {
throw new StackOverflowError();
}
stack.push(Integer.parseInt(command));
}
}
return stack;
}
}
3번
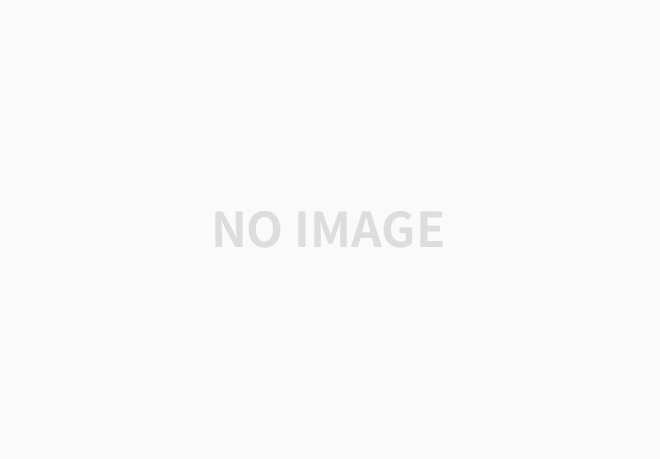
// N개의 정수배열 A와 정수 K가 주어진다.
// 나머지 요소의 진폭이 최소가 되도록 A에서 K개의 연속 요소를 제거하려고 합니다.
// 진폭은 최대 요소와 최소 요소 간의 차이입니다.
// 리턴값은 A에서 K개의 연속적인 요소를 제거한 후 얻을 수 있는 최소 진폭을 나타내는 정수.
// 정수배열에서 최대값과 최소값 차이가 가장적어야함
// 그런데 이제 K만큼 연속적으로 지워야됨(중복아님)
// you can also use imports, for example:
// import java.util.*;
import java.util.Arrays;
// you can write to stdout for debugging purposes, e.g.
// System.out.println("this is a debug message");
class Solution {
public int solution(int[] A, int K) {
int arrayLastIndex = A.length - 1;
Arrays.sort(A);
if (arrayLastIndex == K) {
return 0;
}
int lastMinusFirst = A[arrayLastIndex] - A[0];
int kMinusFirst = A[arrayLastIndex - K] - A[0];
int lastMinusK = A[arrayLastIndex] - A[K];
int[] resultCases = new int[]{lastMinusFirst, kMinusFirst, lastMinusK};
Arrays.sort(resultCases);
return resultCases[0];
}
}
public int solution(int[] A, int K) {
// write your code in Java SE 8
List<Integer> list = Arrays.stream(A).boxed().collect(Collectors.toList());
int result = getAmplitude(copyList(list));
for (int i = 0; i <= list.size() - K; i++) {
List<Integer> copyList = copyList(list);
for (int j = i; j < i+K; j++) {
copyList.remove(i);
}
int amplitude = getAmplitude(copyList);
result = Math.min(result, amplitude);
}
System.out.println(result);
return result;
}
private int getAmplitude(List<Integer> list) {
Collections.sort(list);
int amplitude = list.get(list.size() - 1) - list.get(0);
return amplitude;
}
private List<Integer> copyList(List<Integer> list) {
return list.stream().collect(Collectors.toList());
}
'공부 > 알고리즘' 카테고리의 다른 글
피보나치 수 (프로그래머스 Lv2) - 완료 (0) | 2019.07.20 |
---|---|
행렬의 곱셈 (프로그래머스 Lv2) - 완료 (0) | 2019.07.20 |
Jaden Case 문자열 만들기 (프로그래머스 Lv2) - 완료 (0) | 2019.07.01 |
N개의 최소공배수 (프로그래머스 Lv2) - 완료 (0) | 2019.07.01 |
짝지어 제거하기 (프로그래머스 Lv2) - 완료 (0) | 2019.07.01 |